Table of Contents
VLOOKUP Function in Excel VBA
VLOOKUP is a worksheet function in Excel, but we can also use it in VBA. The functionality of VLOOKUP is similar to the functionality in VBA and worksheets. As it is a worksheet function, the method to use VLOOKUP in VBA is through Application.WorksheetFunction method and the arguments remain the same.
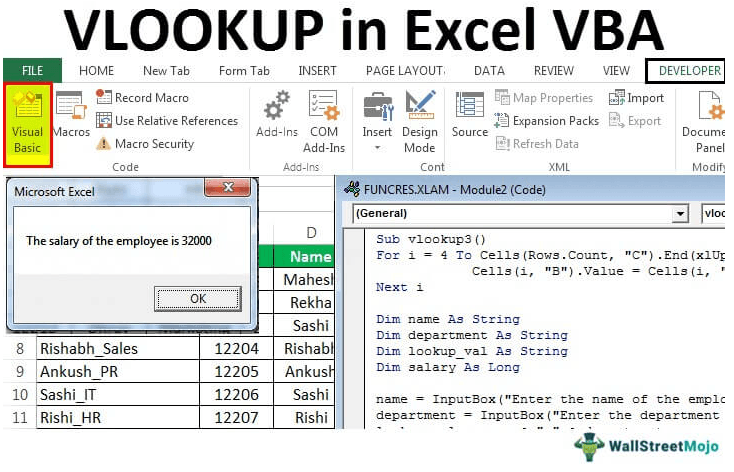
The VLOOKUP function in Excel searches a value in an array and returns its corresponding value from another column. The value to search should be present in the first column. It is also required to mention whether to look for an exact or approximate match. We can use the worksheet function VLOOKUP in VBA coding. However, the function is not built-in VBA. Thus, we can only call using the worksheet.
The VLOOKUP function in Excel has the following syntax:
In which lookup_value is the value to look for, table_arrray is the table, col_index_num is the column number of the return value, and range_lookup signifies if the match is exact or approximate. The range_lookup can be TRUE/FALSE or 0/1.
In VBA code, one can use the VLOOKUP function:
Application.WorksheetFunction.vlookup(lookup_value, table_array, col_index_num, range_lookup)
How to Use VLookup in Excel VBA?
Below are some examples of the VLOOKUP code in Excel VBA.
This topic not only highlights the essential concepts but also provides a broader understanding of the domain. If youâre eager to explore these ideas more thoroughly, this Excel Macros Course offers a structured way to explore further.
VLookup Code in Excel VBA Example #1
Let us see how we can call the worksheet function VLOOKUP in Excel VBA.
Suppose you have the data on studentsâ IDs, names, and average marks.
Now, you want to look up the marks obtained by a student with ID 11004.
To lookup for the value, follow the following steps:
- Go to the âDeveloperâ tab and click on âVisual Basic.â
- Under the VBA window, go to âInsertâ and click on âModule.â
- Now, write the VBA VLOOKUP code. For example, we can use the following VBA VLOOKUP code.
Sub vlookup1()
Dim student_id As Long
Dim marks As Long
student_id = 11004
Set myrange = Range(âB4:D8â)
marks = Application.WorksheetFunction.VLookup(student_id, myrange, 3, False)
End Sub
Firstly, define a student ID, which is the lookup value. Therefore, we define:
student_id = 11004
Next, we define the range in which the value and the return value exist. Since our data is present in the cells B4:D8, we define a range- myrange as:
Set myrange = Range(âB4:D8â)
Finally, we input the VLOOKUP function using the Worksheet function in a variable marks as:
marks = Application.WorksheetFunction.VLookup(student_id, myrange, 3, False)
To print the marks in a message box, let us use the following command:
MsgBox âStudent with ID:â & student_id & â obtained â & marks & â marksâ
It will return:
A student with ID:11004 obtained 85 marks.
Now, click the run button.
You will notice that a message box will appear in the Excel sheet.
VLookup Code in Excel VBA Example #2
Suppose you have the data on the names of employees and their salaries. The data is in columns B and C. Next, you must write a VBA VLOOKUP code, given the employeeâs name in cell F4. It will return the employeeâs salary in cell G4.
Let us write the VBA VLOOKUP code.
1. Define the range in which the values are present, i.e., columns B and C.
Set myrange = Range (âB:Câ)
2. Define the employeeâs name and input the name from cell F4.
Set name = Range (âF4â)
3. Define salary as cell G4.
Set salary = Range (âG4â)
4. Now, call the VLOOKUP function using WorksheetFunction in VBA and input it in salary.Value. It will return the value (output of the VLOOKUP function) in cell G4. We can use the following syntax:
salary.Value = Application.WorksheetFunction.VLookup(name, myrange, 2, False)
5. Now, run the module. Cell G4 will contain the employeeâs salary after you run the VBA VLOOKUP code.
Suppose you change the value of cell F4 to âDavidâ in the worksheet and re-run the code, which will return Davidâs salary.
VLookup Code in Excel VBA Example #3
Suppose you have your companyâs employeesâ data, ID, names, Department, and salary. Using VLOOKUP in VBA, you want to get the salary details of an employee using his name and department.
Since the VLOOKUP function in Excel searches the lookup_value in only a single column, which is the first column of the table_array. It would help if you first made a column containing each employeeâs âNameâ and âDepartment.â
In VBA, let us insert the values âNameâ and âDepartmentâ in column B of the worksheet.
To do this, go to the âDeveloper tabâ and click âVisual Basic.â Then, go to âInsertâ and click on âModuleâ to start a new module.
Let us now write code such that column B contains the values of column D (name) and column E.
The syntax is:
Firstly, use a âforâ loop from i = 4 since the values start from the 4th row in this case. The loop will continue until the end of the last row of column C. So, we can use the variable as a row number inside the âforâ loop.
Then, enter the value to assign for Cell (row_number, column B), which we can give as Cells (i, âBâ).Value, as Cell (row_number, column D) & â_â & Cell (row_number, column E).
Suppose you want to assign the cell B5 = D5 & â_â & E5. Then, you can simply use the code as:
Cells(5, âBâ).Value = Cells(5, âDâ).Value & â_â & Cells(5, âEâ).Value
Now, letâs look for the lookup value in the array B5:E24. You need first to enter the lookup value. Let us take the value (Name and Department) from the user. To do this,
- Define three variables: name, department, and lookup_val as a string.
- Take the name input from the user. Use the code:
name = InputBox (âEnter the name of the employeeâ)
The content in the input box âEnter the ..â will be displayed in the prompt box when you run the code. The string entered in the prompt will be assigned to the name variable.
3. Take the department from the user. We can do this similarly as above.
department = InputBox (âEnter the department of the employeeâ)
4. Assign the name and department with â_â as a separator to the variable lookup_val using the following syntax:
lookup_val = name & â_â & department
5. Write the VLOOKUP syntax to search for the lookup_val in range B5:E24 and return it in a variable salary.
Initialize the variable salary:
Dim salary As Long
Use the VLOOKUP function to find the lookup_val. We can give the table_array as Range(âB:Fâ), and the salary is in the 5th column. We can thus use the following syntax:
salary = Application.WorksheetFunction.VLookup(lookup_val, Range (âB:Fâ), 5, False)
- To print the salary in a message box, use the syntax:
MsgBox (The salary of the employee is â & salary)
Now, run the code. A prompt box will appear in the worksheet where you can enter the name after you enter the name (Say âSashiâ) and click âOK.â
It will open another box in which you can enter the department. After you enter the department, say IT.
It will print the salary of the employee.
If VLOOKUP canât find any employee with the name and department, it will give an error. For example, suppose you give the name âVishnuâ and the department âIT,â it will return âRun-time error â1004â.â
To address this issue, you can specify that on this type of error, print âValue not foundâ instead. To do this,
- Before using the vlookup syntax, use the following code:
On Error GoTo Message
Check:
The trailing code (of Check:) will be monitored, and if it receives an error, it will go to the âmessageâ statement
2. At the end of the code (Before End Sub), specify that if the error number is 1004, then print in the message box, âEmployee data not present.â One can do this using the syntax:
Message:
If Err.Number = 1004 Then
MsgBox (âEmployee data not presentâ)
End If
Module 1:
Sub vlookup3()
For i = 4 To Cells(Rows.Count, âCâ).End(xlUp).Row
Cells(i, âBâ).Value = Cells(i, âDâ).Value & â_â & Cells(i, âEâ).Value
Next iDim name As String
Dim department As String
Dim lookup_val As String
Dim salary As Longname = InputBox(âEnter the name of the employeeâ)
department = InputBox(âEnter the department of the employeeâ)
lookup_val = name & â_â & departmentOn Error GoTo Message
check:
salary = Application.WorksheetFunction.VLookup(lookup_val, Range (âB:Fâ), 5, False)
MsgBox (âThe salary of the employee is â & salary)Message:
If Err.Number = 1004 Then
MsgBox (âEmployee data not presentâ)
End IfEnd Sub
Things to Remember About VLookup in Excel VBA
- The VLOOKUP function can be called in Excel VBA by using WorksheetFunction.
- The syntax of the VLOOKUP function remains the same in Excel VBA.
- When the VBA VLOOKUP code cannot find the lookup_value, it will give a â1004 error.â
- We can manage the error in the VLOOKUP function using a GoTo statement if it returns an error.