Table Of Contents
Excel VBA StrConv Function
The StrConv function in VBA comes under String functions, a conversion function. One may use this function because it changes the case of the string with the input provided by the developer. In addition, the arguments of this function are the string. So, for example, the input for a case like 1 is to change the string to lowercase.
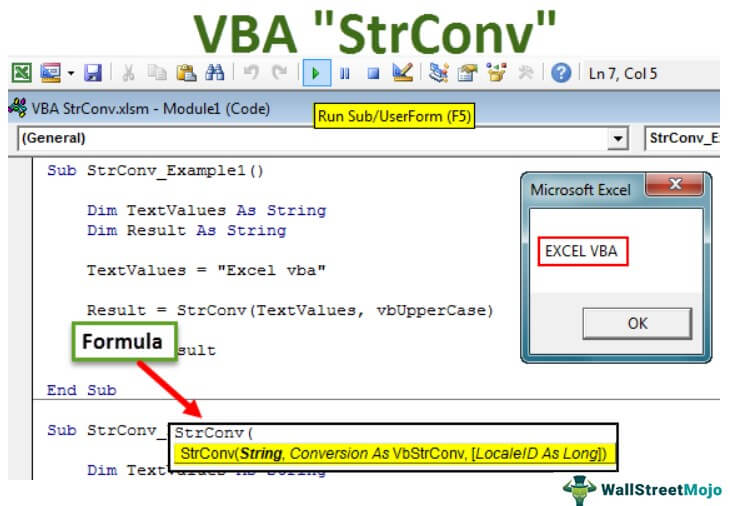
StrConv stands for “String Conversion.” We can convert the supplied string to the specified format using this VBA function. You need to understand that we can use this formula as a VBA function only, not as an Excel worksheet function. This article will tour detailed examples of the “VBA StrConv” formula.
Look at the syntax of the StrConv function.
String: This is nothing but the text we are trying to convert.
Conversion: What kind of conversion do we need to do? We have a wide variety of options. Here, below is the list of conversions we can perform.
- vbUpperCase or 1: This option converts the supplied Text value to upper case character. It works similarly to the UCASE function. For example, if you supply the word “Excel,” it will convert to “EXCEL.”
- vbLowerCase or 2: This option converts the supplied Text value to lowercase character in excel. It works similarly to the LCASE function. For example, if you supply the word “Excel,” it will convert to “excel.”
- vbProperCase or 3: This option converts the supplied Text value to the proper case character. Every first character of the word converts to uppercase. All the remaining letters convert to lowercase. For example, if you supply the word “excEL,” it will convert to “Excel.”
- vbUniCode or 64: This option converts the string to Unicode code.
- vbFromUnicode or 128: It converts the string Unicode to the default system code.
Even though we have several other options with the Conversion argument above, three are good enough for us.
LCID: This is the Locale ID. By default, it takes the system ID. It will not use 99% of the time.
Examples of StrConv Function in VBA
Example #1
Now, look at the example of converting the string to the UPPER CASE character. We are using the word “Excel VBA” here. Below is the VBA code.
Code:
Sub StrConv_Example1() Dim TextValues As String Dim Result As String TextValues = "Excel vba" Result = StrConv(TextValues, vbUpperCase) MsgBox Result End Sub
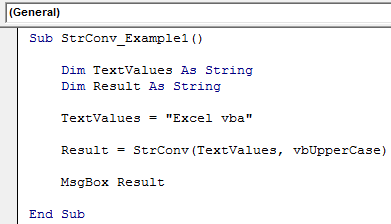
It will convert the string “Excel VBA” to upper case.
Run this code using the F5 key or manually and see the result.
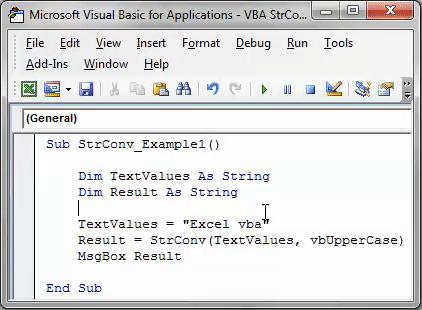
Example #2
Now, take a look at the same string with lowercase conversion. Below is the code.
Code:
Sub StrConv_Example2() Dim TextValues As String Dim Result As String TextValues = "Excel vba" Result = StrConv(TextValues, vbLowerCase) MsgBox Result End Sub
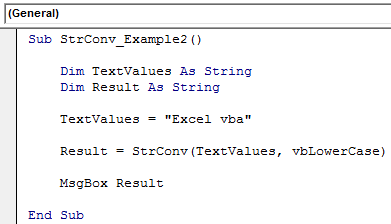
It will convert the string “Excel VBA” to a lowercase.
You can run it manually or through excel shortcut key F5. Below is the result.
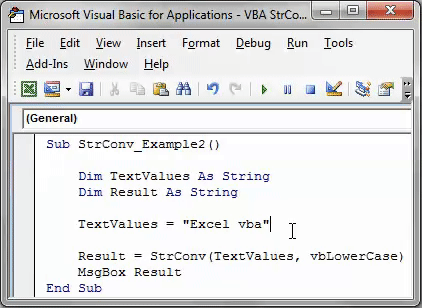
Example #3
Now, take a look at the same string with proper case conversion. Below is the code.
Code:
Sub StrConv_Example3() Dim TextValues As String Dim Result As String TextValues = "Excel vba" Result = StrConv(TextValues, vbProperCase) MsgBox Result End Sub
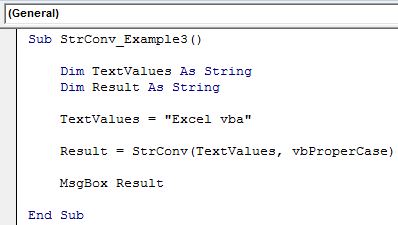
It will convert the string “Excel VBA” to a proper case. Every first letter of the string is upper case. Moreover, it converts every letter after space to uppercase. All the remaining characters convert to lowercase. Below is the result of the same.
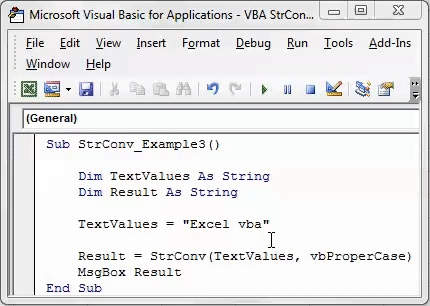
Example #4
Now, look at the Unicode character's example. Look at the below code.
Code:
Sub StrConv_Example4() Dim i As Long Dim x() As Byte x = StrConv("ExcelVBA", vbFromUnicode) For i = 0 To UBound(x) Debug.Print x(i) Next End Sub
It will print all the Unicode characters to the immediate window.
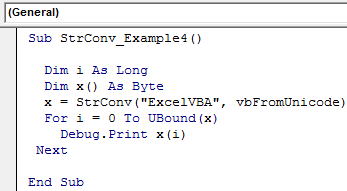
In ASCII code, “E” Unicode is 69, “x” Unicode is 120, and so on. Like this, using VBA StrConv, we can convert the string to Unicode.
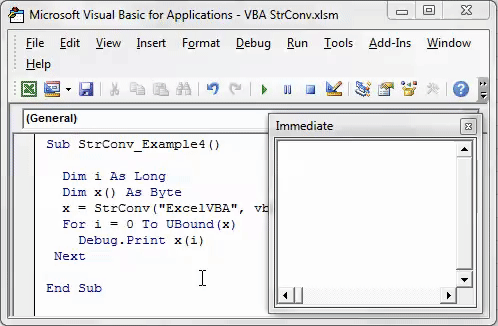