VBA Return
Last Updated :
-
Blog Author :
Edited by :
Reviewed by :
Table Of Contents
Excel VBA Return Statement
VBA is wonderful and a lifesaver for those who know the in and out of VBA coding. It can save tons of time at our workplace. In our earlier articles, we discussed many things and concepts of VBA return. One such concept is the GoSub Return statement. In this article, we will introduce you to these concepts.
Table of contents
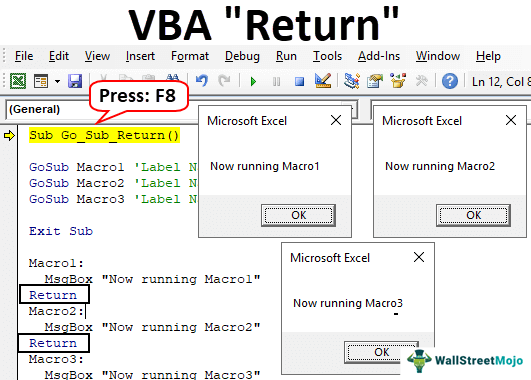
What is a GoSub Return Statement?
The statement "GoSub" means it will go to the line of code indicated by label through name and performs a specific set of the task until it finds the statement "Return."
It is similar to the error handler statement "GoTo Label," but what "GoSub Return" does is return to the next line of code rather than continuing from the label name.
Below is the syntax of the VBA GoSub Return statement.
GoSub
….. Line of Code
:
….. Line of Code to perform the task
We know it is not easy to understand from reading the syntax but using the example, you may be able to understand the concept.
Before we become go-to examples, let me tell you a few things about this statement.
- The GoSub statement calls a subroutine in VBA, named by a label within the same routine or Function.
- Both GoSub and Return should be within the same procedure. This is because we cannot call another subroutine macro here.
- You can introduce any number of GoSub Return statements.
- A return statement will continue the execution of the code from where it left off before it jumps to the sub procedure indicated by a label name.
How to use GoSub Return Statement in VBA?
Example #1
To understand the usage of this statement, look at the below code first. Later, we will explain to you the code line by line.
Code:
Sub Go_Sub_Return() GoSub Macro1 'Label Name1 GoSub Macro2 'Label Name2 GoSub Macro3 'Label Name3 Exit Sub Macro1: MsgBox "Now running Macro1" Return Macro2: MsgBox "Now running Macro2" Return Macro3: MsgBox "Now running Macro3" Return End Sub
Let us run the code by pressing the F8 key to understanding this code. After pressing the F8 key first, it will initiate the macro running.
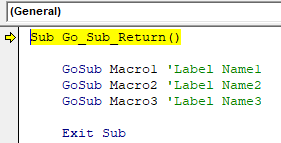
Now, press the F8 key once more to go to the next line.
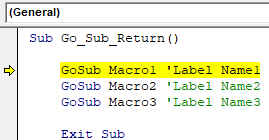
We all know Macro will run the code line by line. But if you press the F8 key, it will not go to the next line. Rather, it works differently.
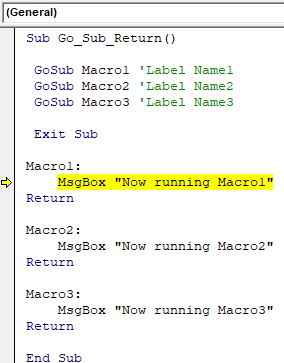
It has jumped to the label named "Macro1" this is because in the previous statement "GoSub," we have instructed the Macro to go to the subprocedure label name "Macro1", so accordingly, it has jumped to the respective label name.
By pressing the F8 key, excel macro will execute the label "Macro1" task of showing the result in the message box.
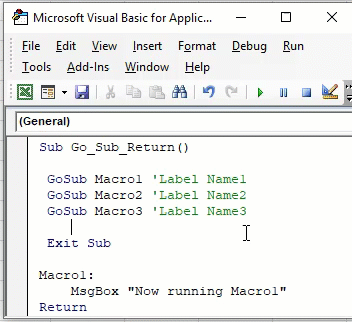
Click on the "OK" message to return to the coding window.
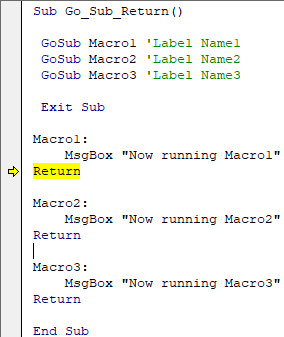
Now, it has highlighted the "Return" statement. So, if you press the F8 key one more time, what it will do is it will "Return Back" to the previous line of code before it jumps to the label name.
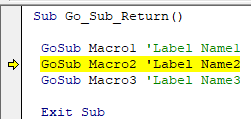
The last time it executed the code "GoSub Macro1," it performed the label name "Macro1" task. Since we have mentioned the "Return" statement, it is back to the next line of code i.e.
“GoSub Macro2”
What the statement says is, "go-to label named Macro2". In the below label "Macro2", we have mentioned a specific set of tasks.
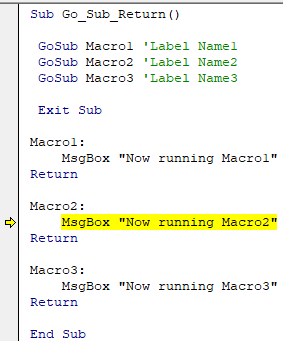
Now, we will get the second subprocedure task of showing value in the message box.
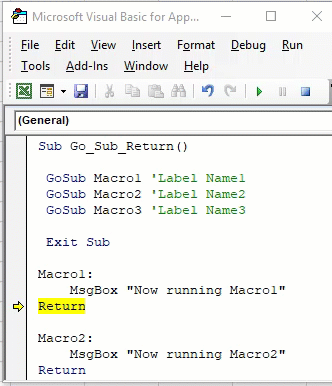
Now, click on "OK." It will highlight the second "Return" statement.
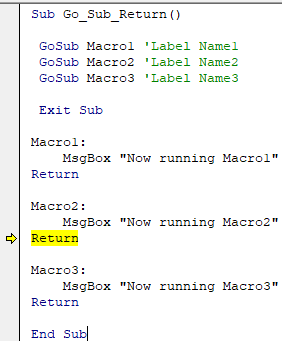
Upon clicking the F8 key, it will return to the next line of code before returning to the label "Macro2". Now, it will highlight "GoSub Macro3."
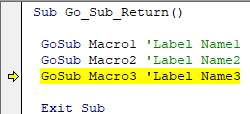
Now, it will go to the label "Macro3" and press the F8 key.
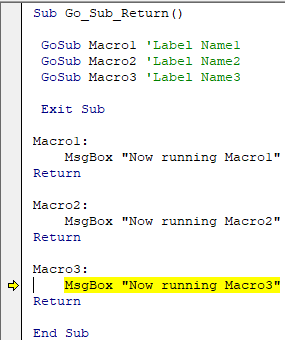
Now, it will execute the third label task.
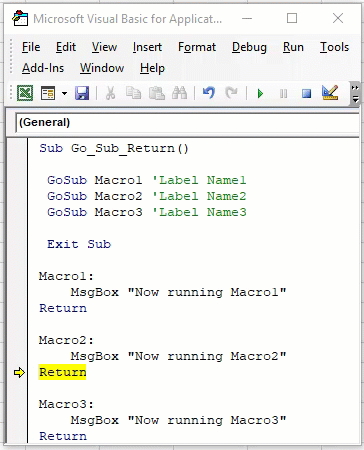
Now, click on "OK." It will highlight the "Return" statement.
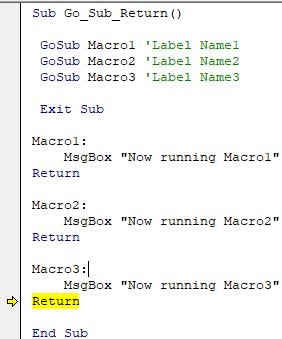
Now, press the F8 key again. It will jump back to the line of code "Exit Sub."
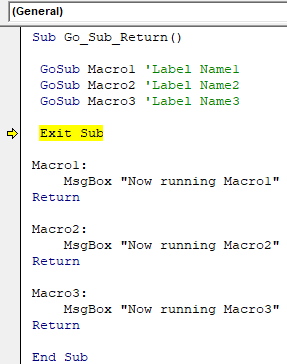
No, it will exit the subroutine. Therefore, it is necessary to add the word "Exit Sub." Otherwise, it will encounter an error.
Example #2
Now, look at one more example of using the GoSub Return statement.
Code:
Sub Go_Sub_Return1() Dim Num As Long Num = Application.InputBox (Prompt:="Please enter the number here", Title:="Divsion Number") If Num > 10 Then GoSub Division Else MsgBox "Number is less than 10" Exit Sub End If Exit Sub Division: MsgBox Num / 5 Return End Sub
This code will ask you to enter the number which is >10. If the number is >10, then it will perform the task of dividing it by 5 (Label Name is "Division"), or else it will show the message as "Number is less than 10."
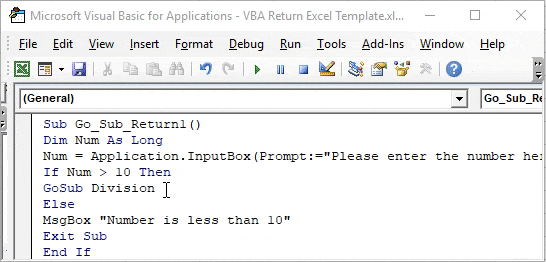
Things to Remember
- The label name should be the same in the GoSub and Return statement, and in the return statement label name should be followed by a colon (:).
- We must always use Exit Sub in VBA after making all the return statements to avoid the error message.
Recommended Articles
This article has been a guide to VBA Return. Here, we learn how to use GoSub Return Statement in VBA, examples, and a downloadable Excel template. Below are some useful Excel articles related to VBA: -