Table Of Contents
Pause VBA Code From Running
VBA Pause one may use to pause the code to execute for a specified amount of time and to pause a code in VBA. We use the application.wait method.
When we build large VBA projects after performing something, we may need to wait for some time to do other tasks. So, how do we pause the macro code to do our task in such scenarios? We can pause the VBA code for a specified period using two functions. Those functions are "Wait" and "Sleep."
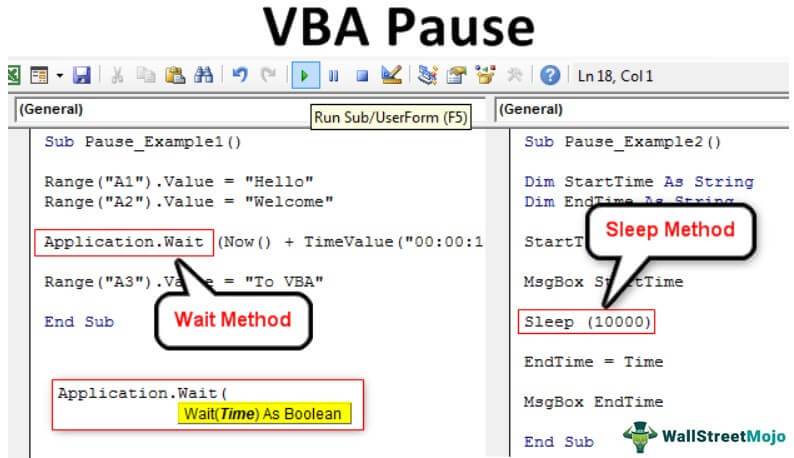
How to Pause Code using the Wait Method?
“Wait” is the function in VBA to hold the macro running for a specific amount of time. So, by applying this function, we need to know when our code should wait.
For example, if executing the code at 13:00:00, if you supply the time as "13:15:00", it will hold the Macro running for 15 minutes.
Now, look at the argument of the WAIT function in VBA.

We must mention when our code should pause or wait in the time argument.
For example, look at the below VBA code.
Code:
Sub Pause_Example1() Range("A1").Value = "Hello" Range("A2").Value = "Welcome" Application.Wait ("13:15:00") Range("A3").Value = "To VBA" End Sub
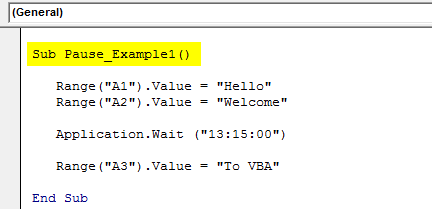
Remember, while running this code, my system time is 13:00:00. As soon as we run the code, it will execute the first two lines i.e.
Range("A1").Value = "Hello” & Range("A2").Value = "Welcome"
But if you look at the next line, it says Application.Wait ("13:15:00"), so after executing those lines tasks, my Macro will be paused for 15 minutes, i.e., from 13:00:00, it will wait until my system time reaches 13:15:01.
Once my system reaches that time, it will execute the remaining lines of code.
Range("A3").Value = "To VBA"
However, this is not the best way of practicing the pause code. For example, let us say you are running the code at different times. Then we need to use the NOW VBA function with TIME VALUE function.
Now function returns the current date & time as per the system we are working on.
TIME Value function holds the time from 00:00:00 to 23:59:29.
Assume we need to pause the code for 10 minutes whenever we run the code. Then, we can use the below code.
Code:
Sub Pause_Example1() Range("A1").Value = "Hello" Range("A2").Value = "Welcome" Application.Wait (Now() + TimeValue("00:00:10")) Range("A3").Value = "To VBA" End Sub
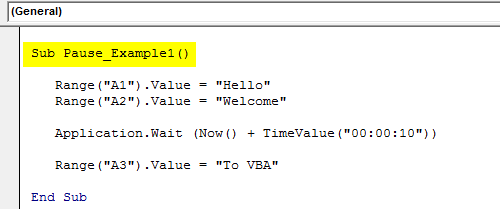
It is similar to the previous code, but the only difference is we have added the NOW and TIME VALUE function.
Whenever we run this code, it will hold or pause the execution for 10 minutes.
How to Pause the VBA Code using the Sleep Method?
Sleep is a complicated function in VBA because it is not a built-in function. Since it is not built-in to make it available to use, we need to add the code below to the top of our module.
Code:
#If VBA7 Then Public Declare PtrSafe Sub Sleep Lib "kernel32" (ByVal dwMilliseconds As LongPtr) 'For 64 Bit Systems #Else Public Declare Sub Sleep Lib "kernel32" (ByVal dwMilliseconds As Long) #End If 'For 32 Bit Systems
You must copy the above code and paste it at the top of the module.
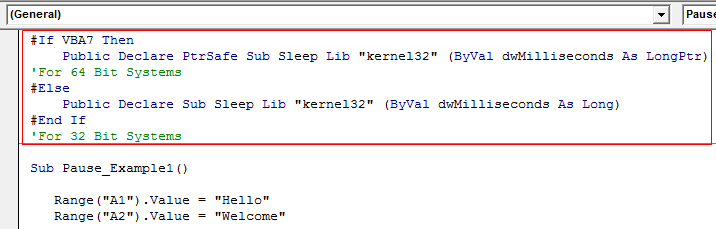
We need to add the above code because SLEEP is a VBA function presented in Windows DLL files, so we need to declare the nomenclature before we start the subprocedure.
Let us look at the example of the SLEEP function now.
Code:
Sub Pause_Example2() Dim StartTime As String Dim EndTime As String StartTime = Time MsgBox StartTime Sleep (10000) EndTime = Time MsgBox EndTime End Sub
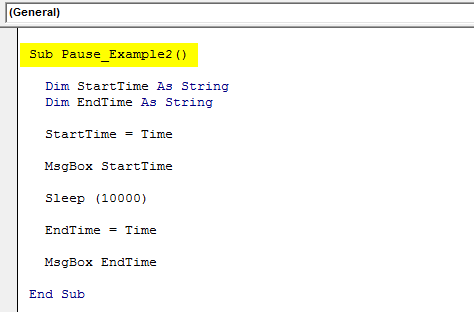
First, we have declared two variables as String.
Dim StartTime As String Dim EndTime As String
Then we assigned the TIME excel function to the StartTime variable. The TIME function returns the current time as per the system.
StartTime = Time
Then we have assigned the same to show in the message box.
MsgBox StartTime
Then, we applied the SLEEP function as Sleep (10000).
Here 10000 is milliseconds, which is equal to 10 seconds in VBA.
Then, we have finally assigned one more TIME function to the variable EndTime.
Now again, we have written a code to show the time.
EndTime = Time
It will show the difference between start time and end time.
We will now execute the code and see the start time.
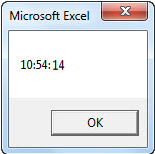
When we execute the code, my system time is 13:40:48. Now, my code will sleep for 10 seconds. So, in the end, my time is as follows.
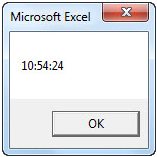
So, like this, we can pause the code from executing it for a specified time.