Table Of Contents
Excel VBA LOOKUP Function
Lookup is the function that helps us to fetch the data from the main table based on a single lookup value. VBA LOOKUP function does not require a data structure like that. For the LOOKUP function, it does not matter whether the result column is to the right or left of the lookup value. Still, it can fetch the data comfortably.
It works similarly to the VLOOKUP function, but more importantly, this is flexible in a data structure. When we say flexible in the data structure, VLOOKUP needs all the values to the right of a lookup value in the main table, and anything left to the lookup value, VLOOKUP cannot find it.
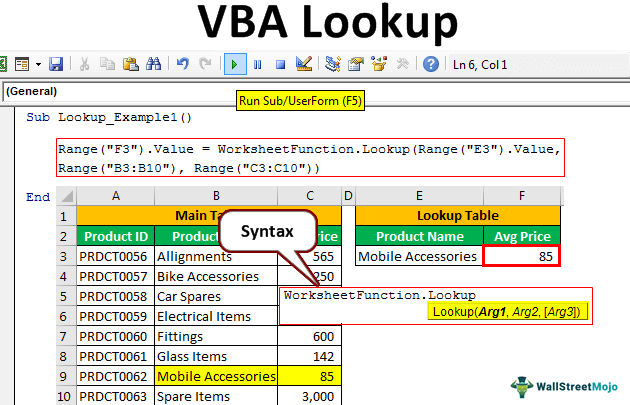
Syntax
If you have already searched for the LOOKUP formula in the VBA editor, we are sure you have gone mad because there is a formula called LOOKUP in VBA.
The reason why it is not available by default is that LOOKUP is not a VBA function. Rather, it is a worksheet function. So to use the LOOKUP function, we must first use the worksheet function in VBA class.
- Arg 1 means lookup value
- Arg 2 is our lookup vector, i.e., the range of our lookup value column
- Arg 3 is our result vector.
How to Use VBA Lookup Function? (Examples)
Example #1
We have the following data from which we want to get the Avg Price of a particular product name using the Lookup function in VBA Excel.
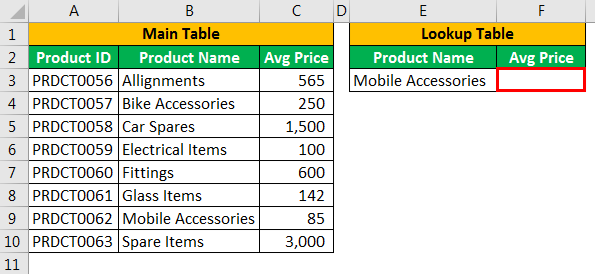
To get the "Avg Price" of "Mobile Accessories," use the following code.
Step 1: Start the subprocedure in VBA.
Code:
Sub Lookup_Example1() End Sub
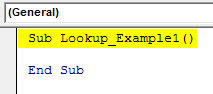
Step 2: We need the result in the F3 cell. So, our code is Range(âF3â).Value =.
Code:
Sub Lookup_Example1() Range("F3").Value End Sub
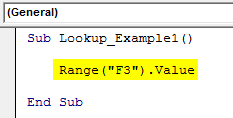
Step 3: As we told you, we could not access the LOOKUP function immediately. So first, use the Worksheet function class.
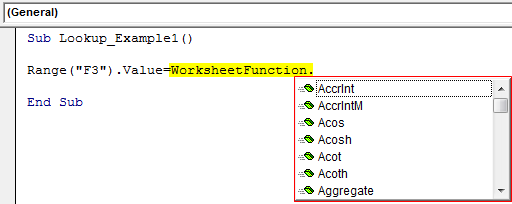
Step 4: The moment we apply the Worksheet function class, we can see all the available formulas with this class in VBA, so select LOOKUP from this list.
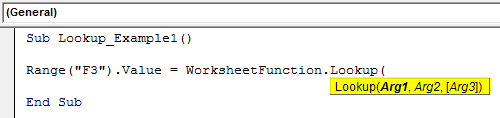
Step 5: With worksheet functions in VBA, we do not see clear arguments of the formula. Here, Arg 1 means lookup value. Our lookup value is in cell E3, so write the code as Range("E3").Value.
Code:
Sub Lookup_Example1() Range("F3").Value = WorksheetFunction.Lookup(Range("E3").Value End Sub
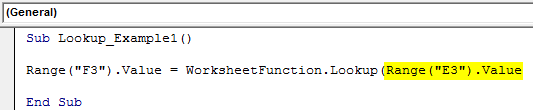
Step 6: Arg 2 is our lookup vector, i.e., the range of our lookup value column. In this example, our lookup vector range is from B3 to B10.
Code:
Sub Lookup_Example1() Range("F3").Value = WorksheetFunction.Lookup(Range("E3").Value,Range("B3:B10"), End Sub

Step 7: Arg 3 is our result vector. We need the result from the Avg Price column, so the result vector is from Range C3 to C10.
Code:
Sub Lookup_Example1() Range("F3").Value = WorksheetFunction.Lookup(Range("E3").Value, Range("B3:B10"), Range("C3:C10")) End Sub
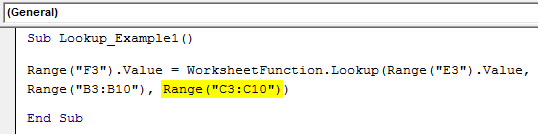
We have completed the VBA coding.
Let us run the code. First, we should get the result in cell F3.
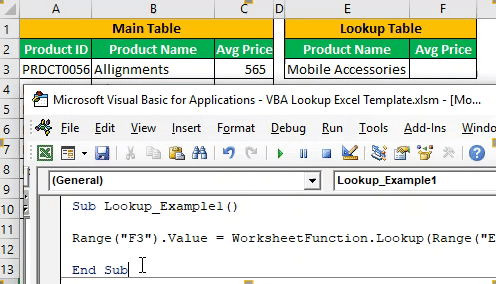
Like this, we can use the LOOKUP function under the worksheet function class to fetch the data of the supplied lookup value.
Example #2
Use Variables for LOOKUP Function
Using variables also, we can arrive at results. Below is the example code of the lookup function with variables.
Code:
Sub Lookup_Example2() Dim ResultCell As Range Dim LookupValueCell As Range Dim LookupVector As Range Dim ResultVector As Range Set ResultCell = Range("F3") Set LookupValueCell = Range("E3") Set LookupVector = Range("B3:B10") Set ResultVector = Range("C3:C10") ResultCell = WorksheetFunction.Lookup(LookupValueCell, LookupVector, ResultVector) End Sub
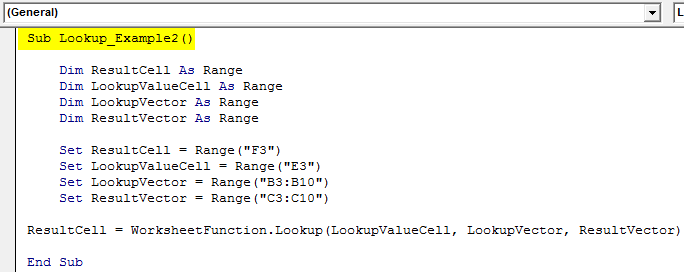
Even though it looks lengthy, it will give good exposure to variables in VBA. Therefore, instead of directly supplying all the values to the formula, we have declared variables and assigned them a set of ranges and values.
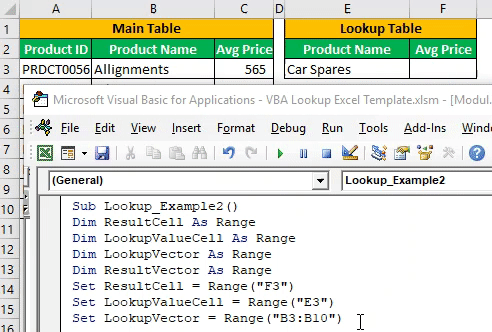
Declaring variables and setting the reference to those variables is always a good practice in any coding language.
Things to Remember
- The LOOKUP function is an alternative function to VLOOKUP.
- The LOOKUP function does not necessarily require the result column to be at the right of the lookup value.
- The range length of both the lookup vector and the result vector should be the same.
- More importantly, we need to supply a column index number, which is necessary for the VLOOKUP function.