Table Of Contents
Excel VBA Last Row
If writing the code is your first progress in VBA, then making the code dynamic is your next step. Excel is full of cell references. The moment we refer to the cell, it becomes fixed. If our data increases, we need to go back to the cell reference and change the references to make the result up to date.
For an example, look at the below code.
Code:
Sub Last_Row_Example1() Range("D2").Value = WorksheetFunction.Sum(Range("B2:B7")) End Sub
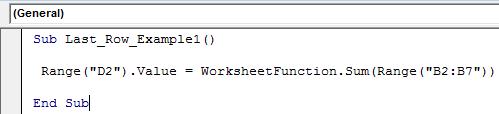
The above code says in D2 cell value should be the summation of Range ("B2:B7").
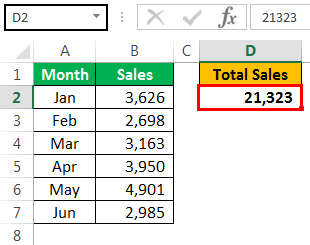
Now, we will add more values to the list.
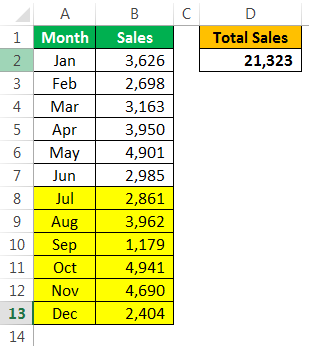
Now, if we run the code, it will not give us the updated result. Rather, it still sticks to the old range, i.e., Range ("B2: B7").
That is where dynamic code is very important.
Finding the last used row in the column is crucial in making the code dynamic. This article will discuss finding the last row in Excel VBA.
How to Find the Last Used Row in the Column?
Below are the examples to find the last used row in Excel VBA.
Method #1
Before we explain the code, we want you to remember how you go to the last row in the normal worksheet.
We will use the shortcut key Ctrl + down arrow.
It will take us to the last used row before any empty cell. We will also use the same VBA method to find the last row.
Step 1: Define the variable as LONG.
Code:
Sub Last_Row_Example2() Dim LR As Long 'For understanding LR = Last Row End Sub
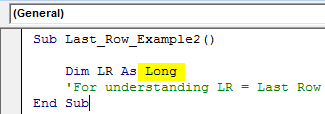
Step 2: We will assign this variable's last used row number.
Code:
Sub Last_Row_Example2() Dim LR As Long 'For understanding LR = Last Row LR = End Sub
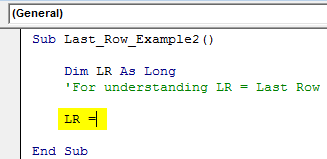
Step 3: Write the code as CELLS (Rows.Count,
Code:
Sub Last_Row_Example2() Dim LR As Long 'For understanding LR = Last Row LR = Cells(Rows.Count, End Sub
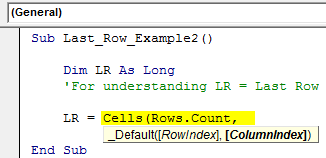
Step 4: Now, mention the column number as 1.
Code:
Sub Last_Row_Example2() Dim LR As Long 'For understanding LR = Last Row LR = Cells(Rows.Count, 1) End Sub
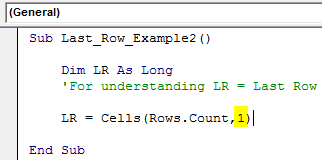
CELLS(Rows.Count, 1) means counting how many rows are in the first column.
So, the above VBA code will take us to the last row of the Excel sheet.
Step 5: If we are in the last cell of the sheet to go to the last used row, we will press the Ctrl + Up Arrow keys.
In VBA, we need to use the end key and up, i.e., BA xlUp
Code:
Sub Last_Row_Example2() Dim LR As Long 'For understanding LR = Last Row LR = Cells(Rows.Count, 1).End(xlUp) End Sub
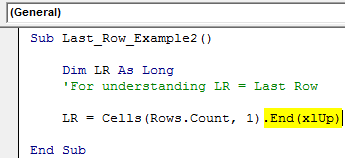
Step 6: It will take us to the last used row from the bottom. Now, we need the row number of this. So, use the property ROW to get the row number.
Code:
Sub Last_Row_Example2() Dim LR As Long 'For understanding LR = Last Row LR = Cells(Rows.Count, 1).End(xlUp).Row End Sub
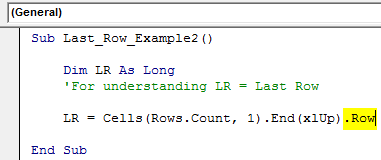
Step 7: Now, the variable holds the last used row number. Show the value of this variable in the message box in the VBA code.
Code:
Sub Last_Row_Example2() Dim LR As Long 'For understanding LR = Last Row LR = Cells(Rows.Count, 1).End(xlUp).Row MsgBox LR End Sub
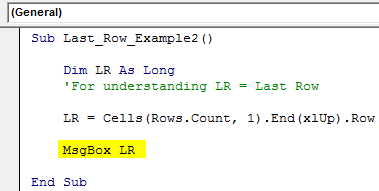
Run this code using the F5 key or manually. It will display the last used row.
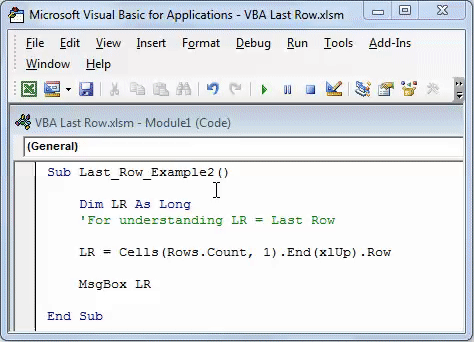
Output:
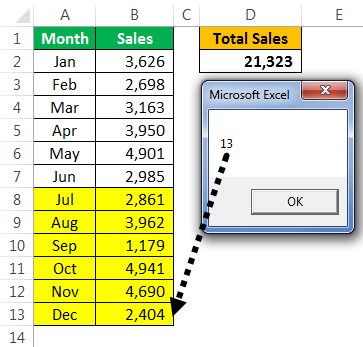
The last used row in this worksheet is 13.
Now, we will delete one more line, run the code, and see the dynamism of the code.
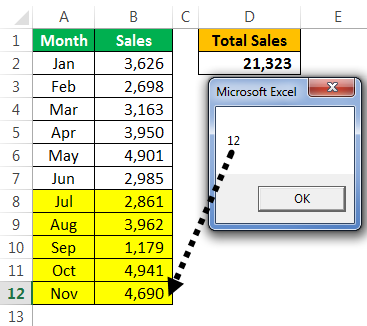
Now, the result automatically takes the last row.
That is what the dynamic VBA last row code is.
As we showed in the earlier example, change the row number from a numeric value to LR.
Code:
Sub Last_Row_Example2() Dim LR As Long 'For understanding LR = Last Row LR = Cells(Rows.Count, 1).End(xlUp).Row Range("D2").Value = WorksheetFunction.Sum(Range("B2:B" & LR)) End Sub
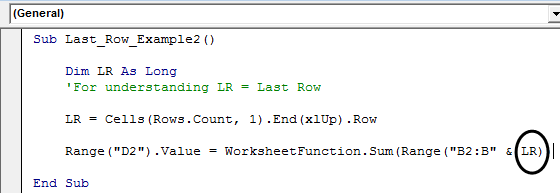
We have removed B13 and added the variable name LR.
Now, it does not matter how many rows you add. It will automatically take the updated reference.
Method #2
We can also find the last row in VBA using the Range object and special VBA cells property.
Code:
Sub Last_Row_Example3() Dim LR As Long LR = Range("A:A").SpecialCells(xlCellTypeLastCell).Row MsgBox LR End Sub
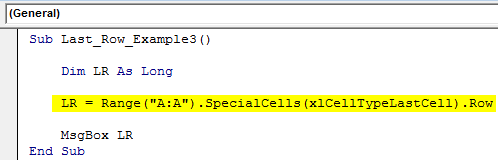
The code also gives you the last used row. For example, look at the below worksheet image.
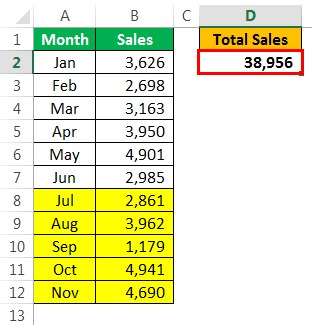
If we run the code manually or use the F5 key result will be 12 because 12 is the last used row.
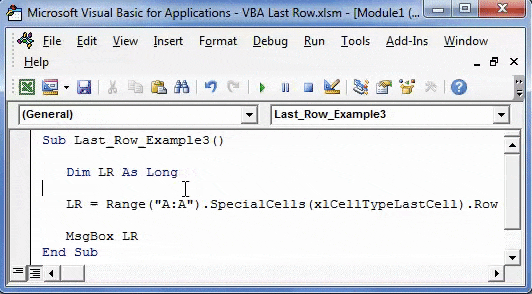
Output:
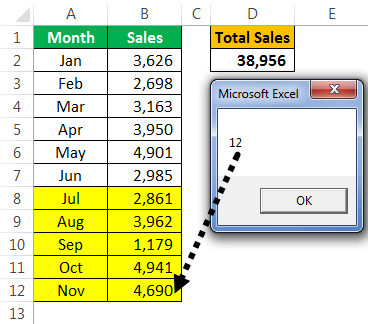
Now, we will delete the 12th row and see the result.
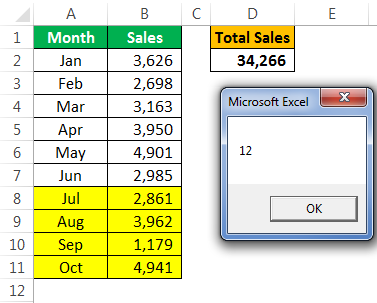
Even though we have deleted one row, it still shows the result as 12.
To make this code work, we must hit the "Save" button after every action. Then this code will return accurate results.
We have saved the workbook and now see the result.
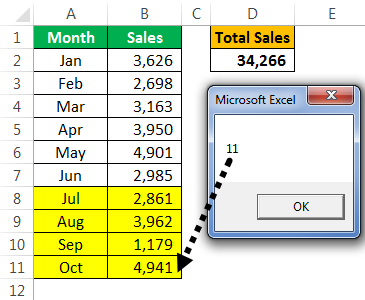
Method #3
We can find the VBA last row in the used range. For example, the below code also returns the last used row.
Code:
Sub Last_Row_Example4() Dim LR As Long LR = ActiveSheet.UsedRange.Rows(ActiveSheet.UsedRange.Rows.Count).Row MsgBox LR End Sub
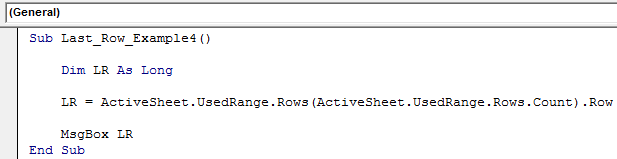
It will also return the last used row.
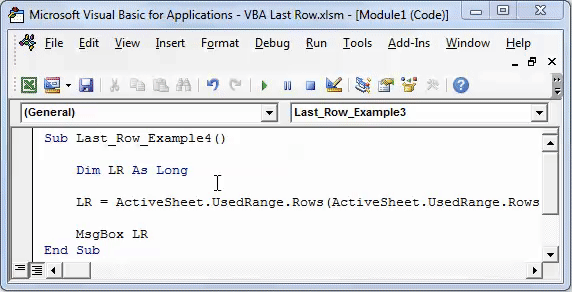
Output:
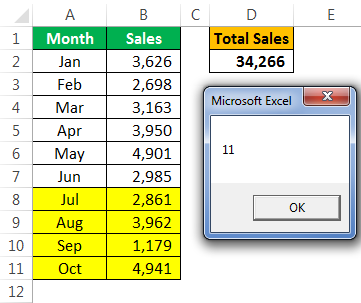