Table Of Contents
Excel VBA JOIN Function
As the name suggests, one may use the VBA JOIN function to combine an array of substrings with the specified delimiter. If we do not specify any delimiter, it takes āspaceā as a default delimiter character. It does the same work as the Concatenate function in Excel, except we only have to specify the delimiter character once. In the Concatenate function, we have to specify the delimiter character every time between every two strings.
The syntax of the function is
As we can see, the function takes two arguments and returns a string. Arguments are:
- SourceArray: We need to specify or reference an array of substrings joined.
- Delimiter: The delimiter is used to separate each substring when creating the resultant string. As this is an optional argument, if we omit it, the delimiter is set to be a space ā ā.
The VBA SPLIT function is the opposite of the VBA JOIN function.
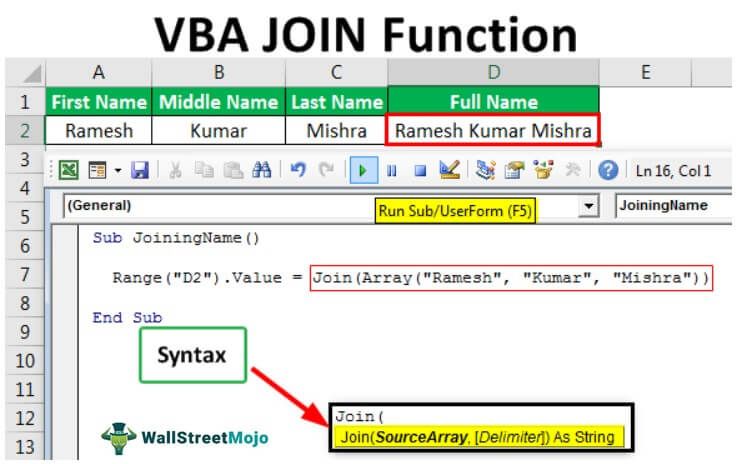
Examples of VBA Join Function
Below are examples of the Join function in Excel VBA.
VBA Join - Example #1
Suppose we want to join the first (Ramesh), middle (Kumar), and last name (Mishra).

Steps would be:
- First, we need to open the Visual Basic Editor. We can do the same by clicking on the āVisual Basicā command in the āCodeā group under the 'Developer' tab excel or using use the excel shortcut key Alt+F11.
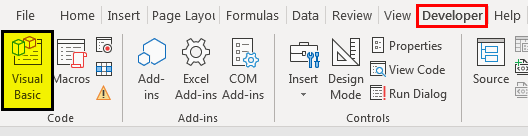
- Insert the module by right-clicking on āsheet 1ā and choosing the āInsertā command from the contextual menu, and then choose āModuleā to insert.
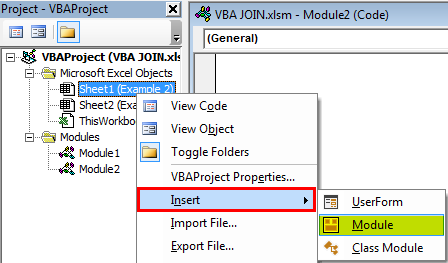
- Create a subroutine named āJoiningNameā.
Code:
Sub JoiningName() End Sub
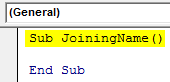
- Use the JOIN function as follows.
Code:
Sub JoiningName() Range("D2").Value = Join(Array("Ramesh", "Kumar", "Mishra")) End Sub
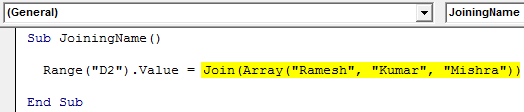
We can see that we have used the ARRAY function to provide SourceArray to the JOIN function and skipped to specify the delimiter character, so āspaceā would be the default character. The processed value of the JOIN function will be written in cell D2 when we execute this code using the F5 key or manually.
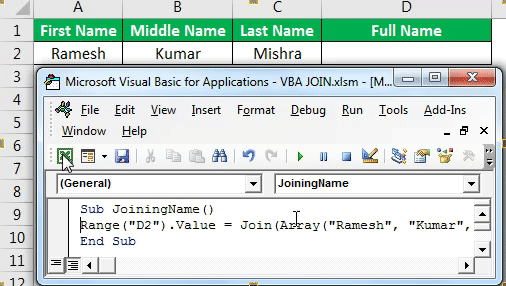
VBA Join - Example #2
Suppose we want to create various Excel files with the item name containing sales only for that item.
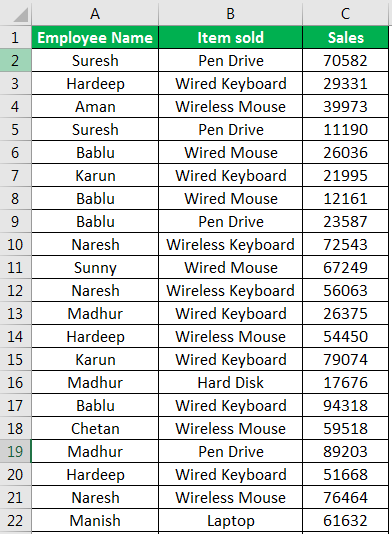
- Open the Visual Basic Editor using the shortcut key Alt+F11.
- Right-click on the āSheet1ā² (Example 2)ā sheet to open the contextual menu and click on āInsertā to insert a VBA 'Module' in the VBA project.
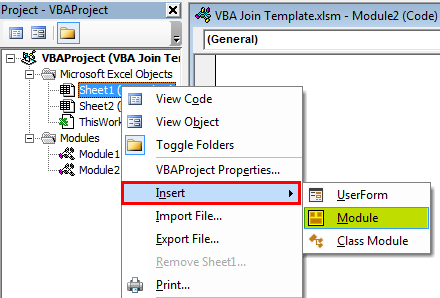
- Define a subroutine named āCreateItemSoldFilesā.
Code:
Sub CreateItemSoldFiles() End Sub
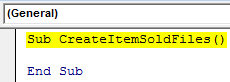
- We need to set a reference to the āMicrosoft Scripting Runtimeā object library using the Tools menu -> References⦠command, as we will use some code (objects), which will not work if we do not include this object library.
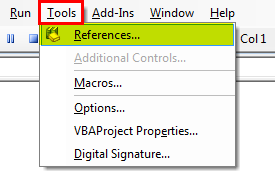
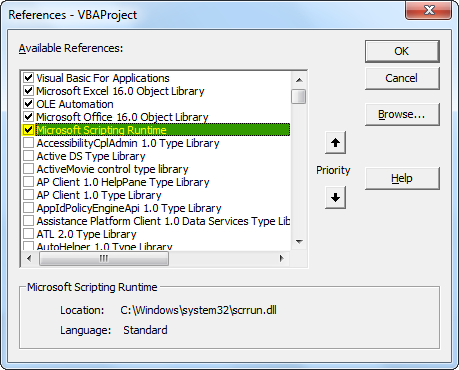
- Now, we will declare all the variables.
Code:
Dim FSO As New Scripting.FileSystemObject
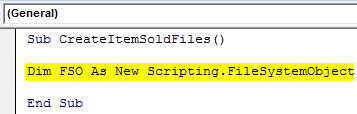
The above FSO variable gives access to the VBA FileSystemObject. After binding, we can use functions like BuildPath, CopyFile, CreateTextFile, etc.
- The next statement creates a TextStream object. Through the TextStream object, we can read from or append to the original file.
Code:
Dim FSO As New Scripting.FileSystemObject Dim ts As Scripting.TextStream
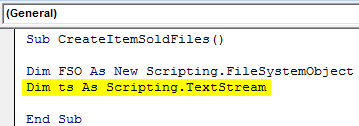
- We will declare more variables. ārā is for holding rows in the range, āfsā is for storing the final joined string, ācolsā for storing numbers of columns in the range, āFolPathā for storing the path of the folder so that we can save the files in the folder and āItems_Soldā for storing various item names to create a file with these names.
Code:
Dim r As Range Dim fs As String Dim cols As Integer Dim FolPath As String Dim Items_Sold As String
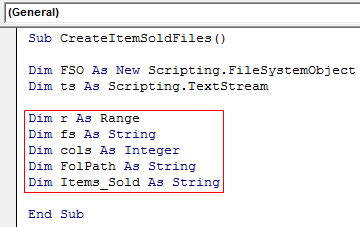
- We will define the following statement to count the total number of columns in the range.
Code:
cols = Range("A1").CurrentRegion.Columns.Count
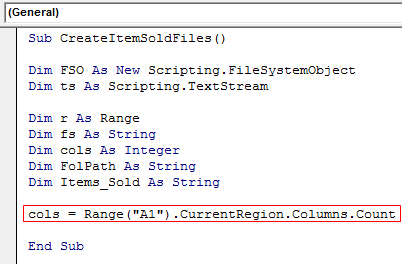
This statement will first select the current region for cell A1 and then count the total number of columns in the current region.
- We will write the following statements for assigning the variable āFolPathā a path using the VBA ENVIRON function and Concatenation Operator.
Code:
FolPath = Environ("UserProfile") & "DesktopItems_Sold" If Not FSO.FolderExists(FolPath) Then FSO.CreateFolder FolPath
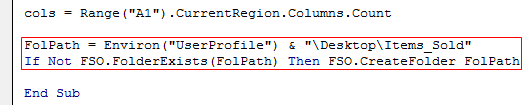
The second statement will create the folder if the folder does not exist in the same location.
- This code will assign the values of the B column one by one to āItems_Sold.ā We have used the āOFFSET functionā to get the reference of the cell in the B column as the currently selected cell is in column A.
Code:
Items_Sold = r.Offset(0, 1).Value
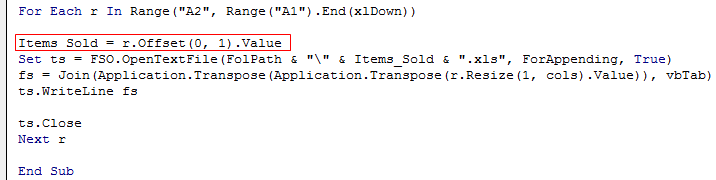
- The following bordered statement will open the files with names stored in the āItems_Soldā variable one by one in appending mode (it will append the new values at last).
Code:
Set ts = FSO.OpenTextFile(FolPath & "" & Items_Sold & ".xls", ForAppending, True)
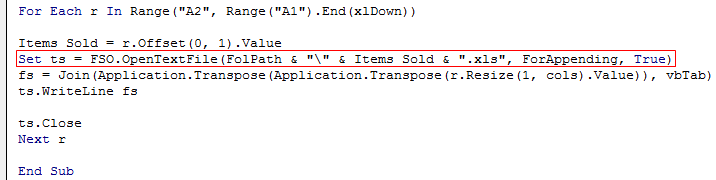
We have used the Concatenate operator with variables āFolPathā and āItems_Soldā and static values (āā andā.xlsā) to create file names for excel files.
- We need to remember that the VBA JOIN function takes only a one-dimensional array as SourceArray To convert the rows into a one-dimensional array, we need to use Application.Transpose method two times.
Code:
fs = Join(Application.Transpose(Application.Transpose(r.Resize(1, cols).Value)), vbTab)
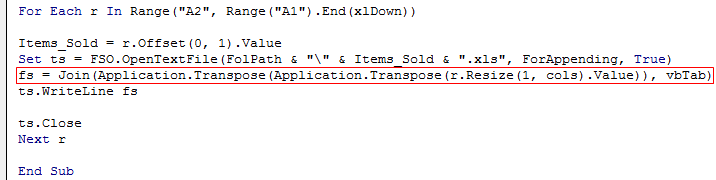
We have used the Resize method of range object to resize the range to the width of several columns in the range.
As a delimiter, we have used the āvbTabā keyword so that it would fill values in different cells.
- As we have stored the processed value of the JOIN function into the āfsā variable, we will write the fsās values into new lines of VBA created Excel files for every row in our original file from row number 2 to the last row (in our case it is 350th row).
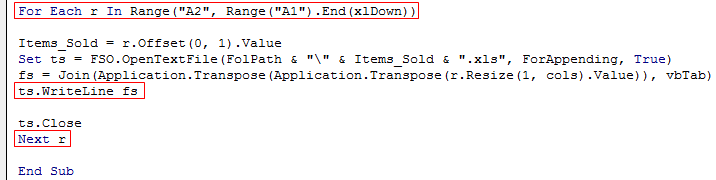
- Before ending the loop, we will close the file. The code would be as shown in the screenshot.
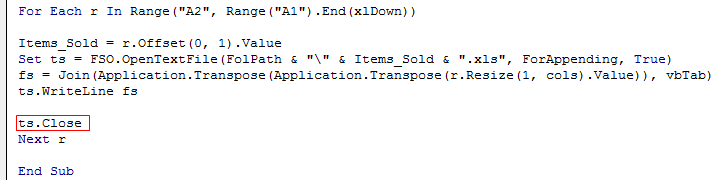
We have written the full code now.
Code:
Sub CreateItemSoldFiles() Dim FSO As New Scripting.FileSystemObject Dim ts As Scripting.TextStream Dim r As Range Dim fs As String Dim cols As Integer Dim FolPath As String Dim Items_Sold As String cols = Range("A1").CurrentRegion.Columns.Count FolPath = Environ("UserProfile") & "DesktopItems_Sold" If Not FSO.FolderExists(FolPath) Then FSO.CreateFolder FolPath For Each r In Range("A2", Range("A1").End(xlDown)) Items_Sold = r.Offset(0, 1).Value Set ts = FSO.OpenTextFile(FolPath & "" & Items_Sold & ".xls", ForAppending, True) fs = Join(Application.Transpose(Application.Transpose(r.Resize(1, cols).Value)), vbTab) ts.WriteLine fs ts.Close Next r End Sub
To execute the code, we will press F5. Then, we can see that it created a folder named āItems_Soldā with the help of VBA code on the desktop.
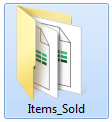
In the folder, there are 7 unique files created with the names of the item. Therefore, we can find details about only that particular item in files.
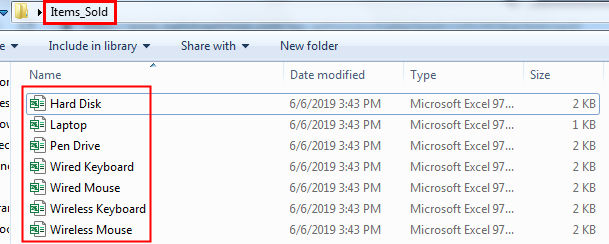
Laptop.xls
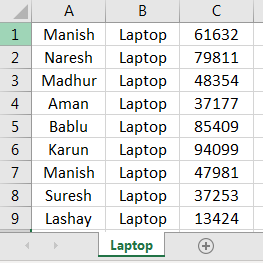
Things to Remember About VBA JOIN Function
- The SourceArray must be a one-dimensional array. Therefore, we cannot refer to an individual cell, as this will create multiple multi-dimensional arrays.
- Suppose we specify a zero-length string (āā) as a delimiter, all items in the array concatenated with no delimiters.