Table Of Contents
IF NOT in VBA
Logical functions are useful for calculations that require multiple conditions or criteria to test. In our earlier articles, we have seen "VBA IF," "VBA OR," and "VBA AND" conditions. This article will discuss the "VBA IF NOT" function. Before introducing VBA IF NOT function, let me show you about VBA NOT function first.
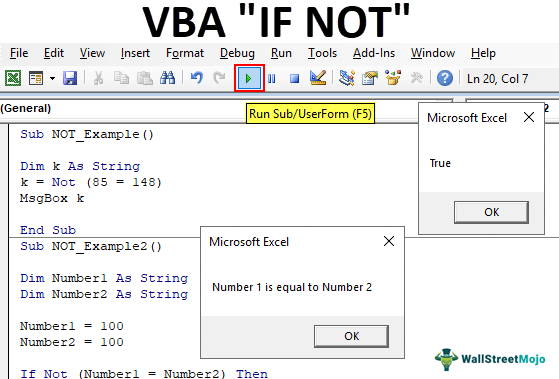
What is NOT Function in VBA?
The "NOT" function is one of our logical functions with Excel and VBA. All the logical functions require logical tests to perform and return TRUE if the logical test is correct. If the logical test is incorrect, it will return FALSE.
But "VBA NOT" is the opposite of the other logical function. So, we would say this is the inverse function of logical functions.
The "VBA NOT" function returns "FALSE" if the logical test is correct. If the logical test is incorrect, it will return "TRUE." Now, look at the syntax of the "VBA NOT" function.
NOT(Logical Test)
It is very simple. First, we need to provide a logical test. Then, the NOT function evaluates the test and returns the result.
Examples of NOT & IF Function in VBA?
Below are the examples of using the IF and NOT function in excel VBA.
Example #1
Take a look at the below code for an example.
Code:
Sub NOT_Example() Dim k As String k = Not (100 = 100) MsgBox k End Sub
In the above code, we have declared the variable as a string.
Dim k As String
Then, for this variable, we have assigned the NOT function with the logical test as 100 = 100.
k = Not (100 = 100)
Then, we have written the code to show the result in the VBA message box. MsgBox k
Now, we will execute the code and see the result.

We got the result as "FALSE."
Now, look back at the logical test. We have provided the logical test as 100 = 100, which is generally TRUE; since we had given the NOT function, we got the result as FALSE. As we said in the beginning, it gives inverse results compared to other logical functions. Since 100 equals 100, it has returned the result as FALSE.
Example #2
Now, we will look at one more example with different numbers.
Code:
Sub NOT_Example() Dim k As String k = Not (85 = 148) MsgBox k End Sub
The code is the same. The only thing we have changed here is We have changed the logical test from 100 = 100 to 85 = 148.
Now, we will run the code and see what the result is.

This time we got the result as TRUE. Now, examine the logical test.
k = Not (85 = 148)
We all know 85 is not equal to the number 148. Since it is not equal, the NOT function has returned the result as TRUE.
NOT with IF Condition:
In Excel or VBA, logical conditions are incomplete without the combination IF condition. Using the IF condition in excel we can do many more things beyond default TRUE or FALSE. For example, we got FALSE and TRUE default results in the above examples. Instead, we can modify the result in our own words.
Look at the below code.
Code:
Sub NOT_Example2() Dim Number1 As String Dim Number2 As String Number1 = 100 Number2 = 100 If Not (Number1 = Number2) Then MsgBox "Number 1 is not equal to Number 2" Else MsgBox "Number 1 is equal to Number 2" End If End Sub
We have declared two variables.
Dim Number1 As String & Dim Number2 As String
For these two variables, we have assigned the numbers 100 and 100, respectively.
Number1 = 100 & Number2 = 100
Then, we have attached the IF condition to alter the default TRUE or FALSE for the NOT function. If the result of the NOT function is TRUE, then my result will be as follows.
MsgBox "Number 1 is not equal to Number 2."
If the NOT function result is FALSE, my result is as follows.
MsgBox "Number 1 is equal to Number 2."
Now, we will run the code and see what happens.

We got the result as "Number 1 is equal to Number 2", so the NOT function has returned the FALSE result to the IF condition. So, the IF condition returned this result.
Like this, we can use the IF condition to do the inverse test.