Table Of Contents
Excel VBA Boolean Operator
Boolean is a data type. It is also a built-in data type in VBA. This data type is used for logical references or logical variables because the value this data type holds is either TRUE or FALSE, used for logical comparison. The declaration of this data type is similar to all the other data types.
As we said, the Boolean data type can hold either TRUE or FALSE as the data, but it can also hold number 1 as TRUE and 0 as FALSE. So, TRUE is represented by 1, and FALSE is represented by 0. So, when we declare the variable as BOOLEAN, it occupies 2 bytes of computer memory.
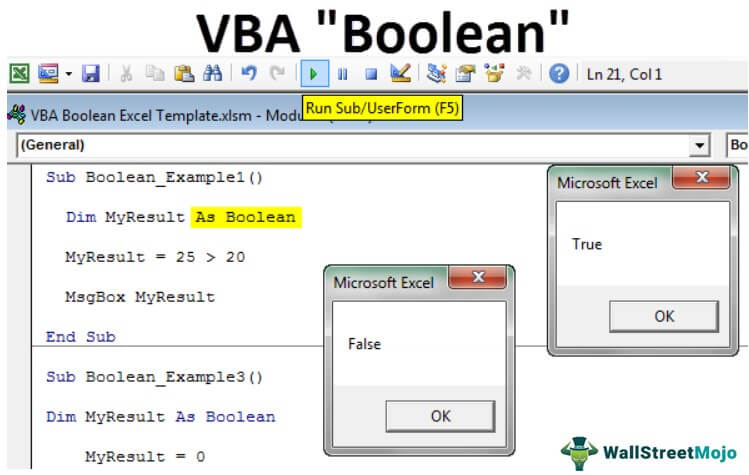
Working with Boolean Data Type in VBA Programming Language
Let us see the example of setting Boolean operator values to variables using the VBA Code.
Follow the steps below to gain adequate knowledge of Boolean data types in VBA.
Step 1: First, start the subprocedure by naming the macro name.
Code:
Sub Boolean_Example1() End Sub
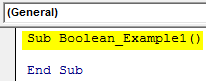
Step 2: Declare the variable as “BOOLEAN.”
Code:
Sub Boolean_Example1() Dim MyResult As Boolean End Sub
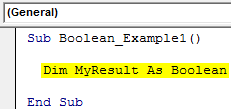
Step 3: Now, for the variable “MyResult,” apply the simple logical test as 25 > 20.
Code:
Sub Boolean_Example1() Dim MyResult As Boolean MyResult = 25 > 20 End Sub
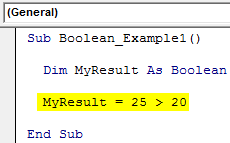
Step 4: Now, show the result in a message box in VBA.
Code:
Sub Boolean_Example1() Dim MyResult As Boolean MyResult = 25 > 20 MsgBox MyResult End Sub
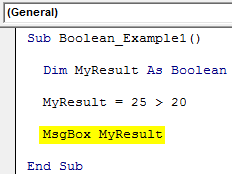
Now, run the excel macro through the F5 key or manually and see the result.
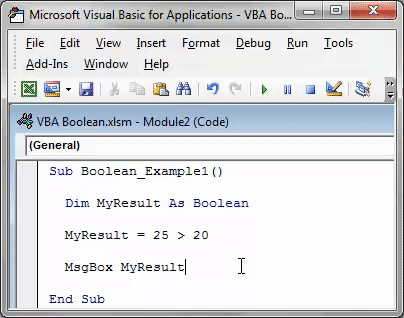
We got the result as TRUE because the number 25 is greater than the number 20, so the logical test is correct, and the result is TRUE.
It is the basic structure of VBA Boolean data types.
Boolean Data Type Cannot Hold Other than TRUE or FALSE.
VBA Boolean is a logical data type. It holds either TRUE or FALSE. Anything other than TRUE or FALSE will show an error message as “Type Mismatch” in VBA.
For example, look at the below code.
Code:
Sub Boolean_Example2() Dim BooleanResult As Boolean BooleanResult = "Hello" MsgBox BooleanResult End Sub
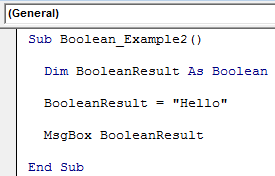
In the above code, we have declared the variable “BooleanResult” as Boolean.
Dim BooleanResult As Boolean
In the next line, we have assigned the value to the declared variable as “Hello.”
BooleanResult = "Hello"
We have declared the variable as Boolean, but we have assigned the value as “Hello,” which is other than logical values: TRUE or FALSE.
When we run this code using the F5 key or manually, we will get the type mismatch error because of the data type mismatch value.
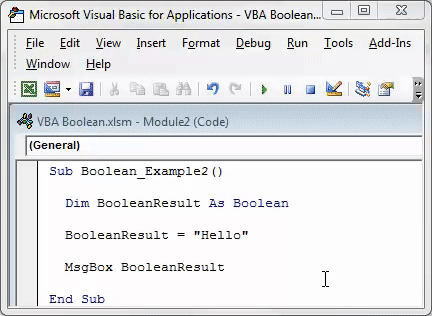
All the Numbers are TRUE, and Zero is FALSE
As we said, TRUE is represented by the number 1, and FALSE is represented by 0. For example, look at the below code in VBA.
Code:
Sub Boolean_Example3() Dim BooleanResult As Boolean BooleanResult = 1 MsgBox BooleanResult End Sub
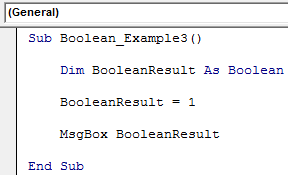
We assigned the value to the variable as 1, showing the result as TRUE.
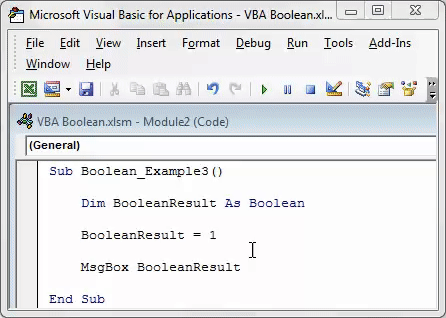
Now, look at the code below.
Code:
Sub Boolean_Example3() Dim BooleanResult As Boolean BooleanResult = 0 MsgBox BooleanResult End Sub
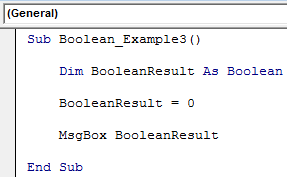
In this code, we have assigned the value to the variable as 0, showing the result as FALSE.
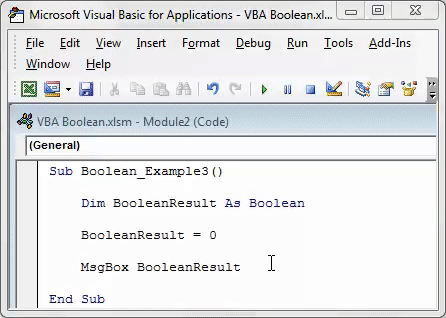
Not only 1 or 0, but it can also treat any number assigned to the variable except zero as TRUE. It will only treat zero as 1.
VBA Boolean Operator with IF Condition
Since the Boolean data type can hold only logical values, it is best suited to use with the IF condition in VBA.
Code:
Sub Boolean_Example2() Dim Number1 As Integer Dim Number2 As Integer Number1 = 80 Number2 = 75 If Number1 >= Number2 Then MsgBox True Else MsgBox False End If End Sub
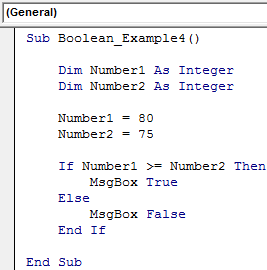
Like this, we can use Excel VBA Boolean data types to store the results as either TRUE or FALSE.